引言
二叉查找树的定义:左孩子比父节点小,右孩子比父节点大的二叉树。
显然,通过中序遍历可得到从小到大的序列,因而二叉查找树常用于快速查找,排序等场合。
下图就是一棵典型的二叉查找树:
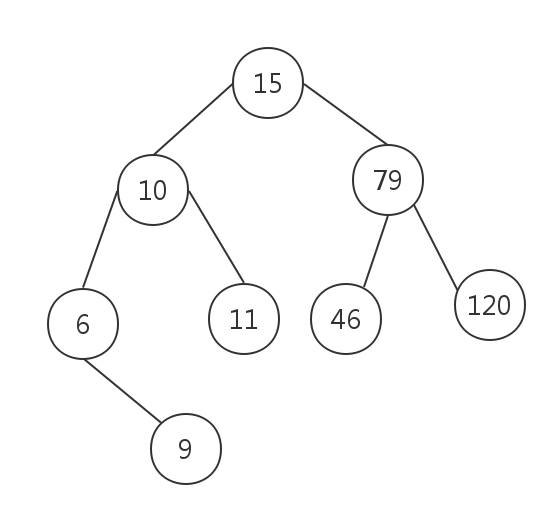
1.二叉查找树的创建
二叉查找树的定义如下:
|
|
利用二叉查找树的定义即可得到其节点插入方法.代码如下:
|
|
插入方法有了之后,二叉树的创建方法就简单了:
|
|
2.包含
判断是否包含的方法很简单:如果小于当前节点,则跟其左子树比较;如果大于当前节点,则与其右子树比较;若等于,则返回true;
|
|
3.查找最小和最大节点
|
|
4.寻找前驱和后驱节点
前驱节点:二叉树中左子树的最大节点;
后驱节点:二叉树中右子树的最小节点;
显然,中序遍历时它们分别排在根节点的前面和后面.
由于上面已经给出了findMax()和findMin的方法,从而查找前驱和后驱节点的方法也非常简单:
|
|
值得注意的是,前驱节点和后驱节点都最多只有一个孩子:前驱节点最多只有一个右孩子,后驱节点最多只有一个左孩子.
后面删除节点时会用到这个性质.
5.移除节点
删除较为复杂,主要考虑以下情况:
- 1)无孩子
直接移除 - 2)单孩子
这个比较简单,如果删除的节点有左孩子那就把左孩子顶上去,如果有右孩子就把右孩子顶上去,如下图所示:
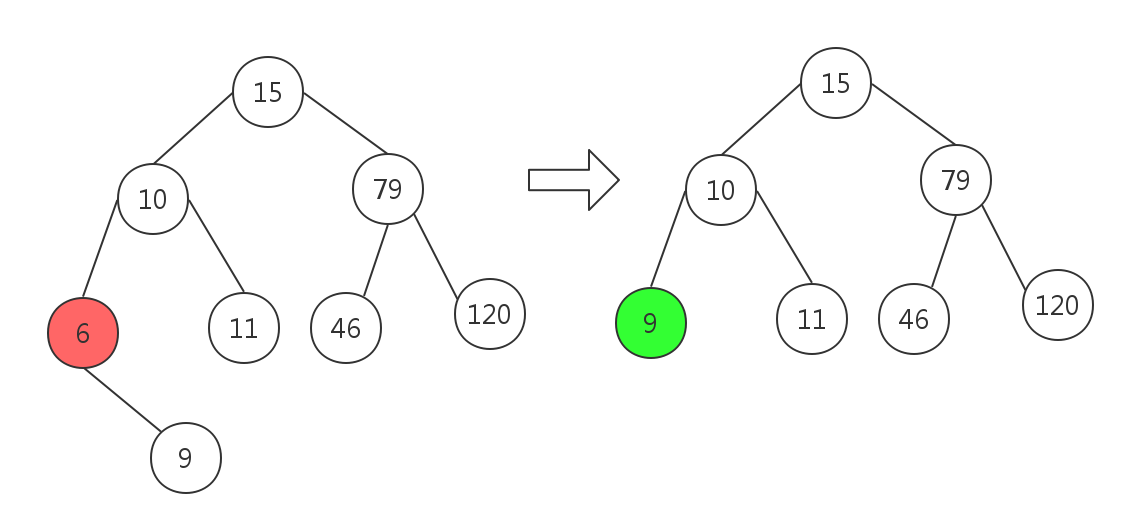
- 3)双孩子
对于一个数组,如果我们要删除一个元素,那么可以让其前面或后面的元素来顶替它.如下图所示:
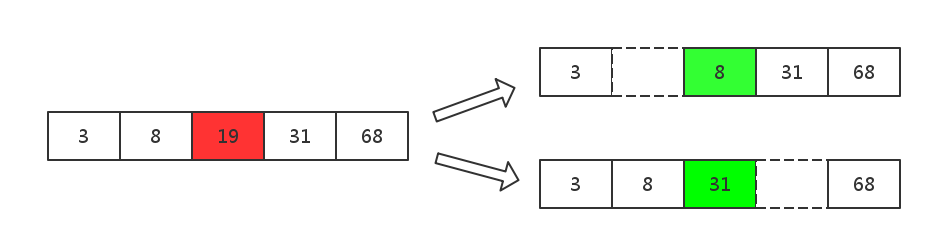
显然,这个要利用前面说的前驱和后驱节点,即既可以利用其前驱节点来替换它,也可以利用其后驱节点来替换它.如下图所示:
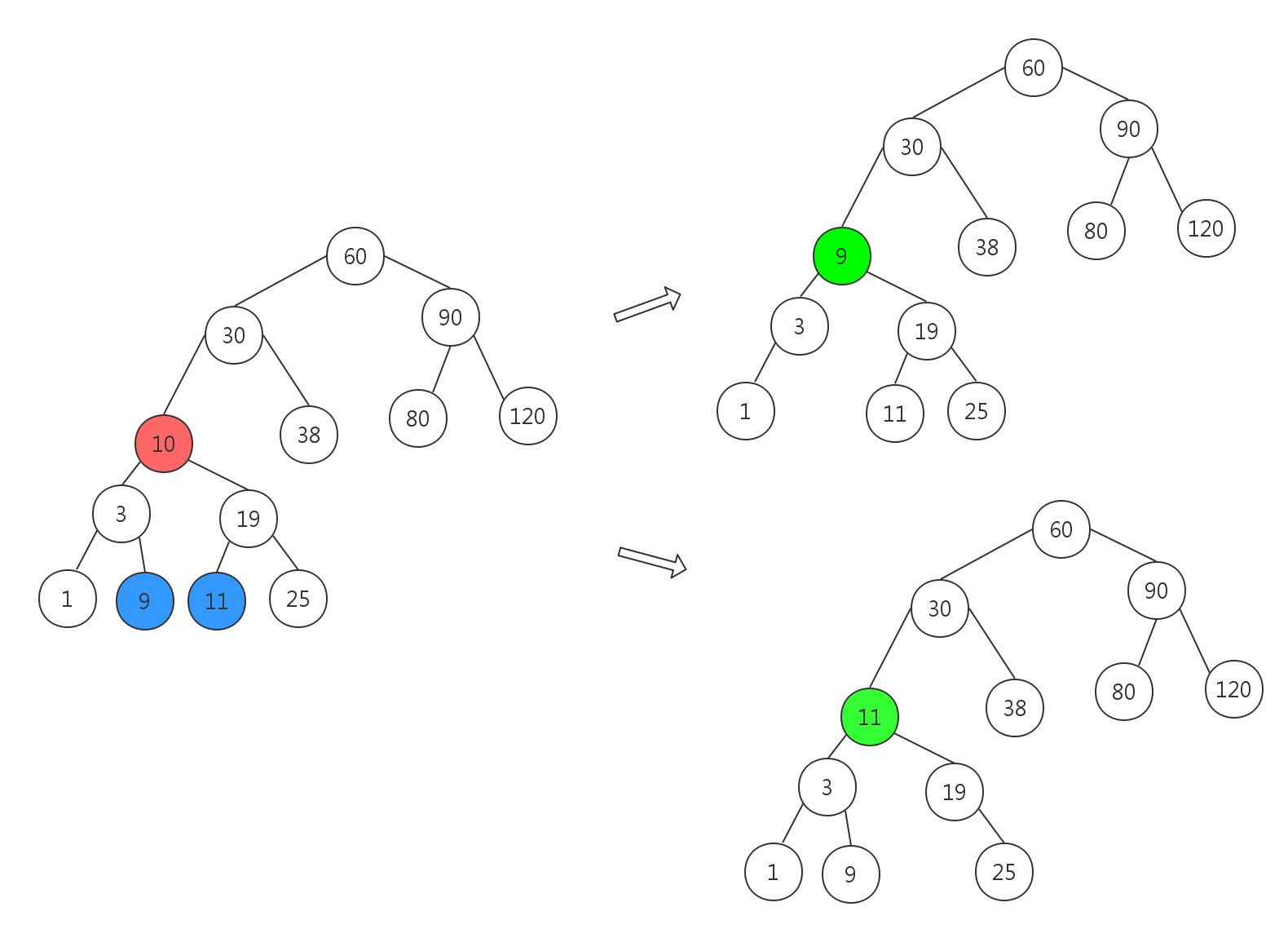
|
|
上面对于左右孩子都不为空的情况利用了前驱节点,其实也可以利用后驱节点,代码片段如下:
|
|
6.测试
测试源码如下:
|
|
测试结果如下:
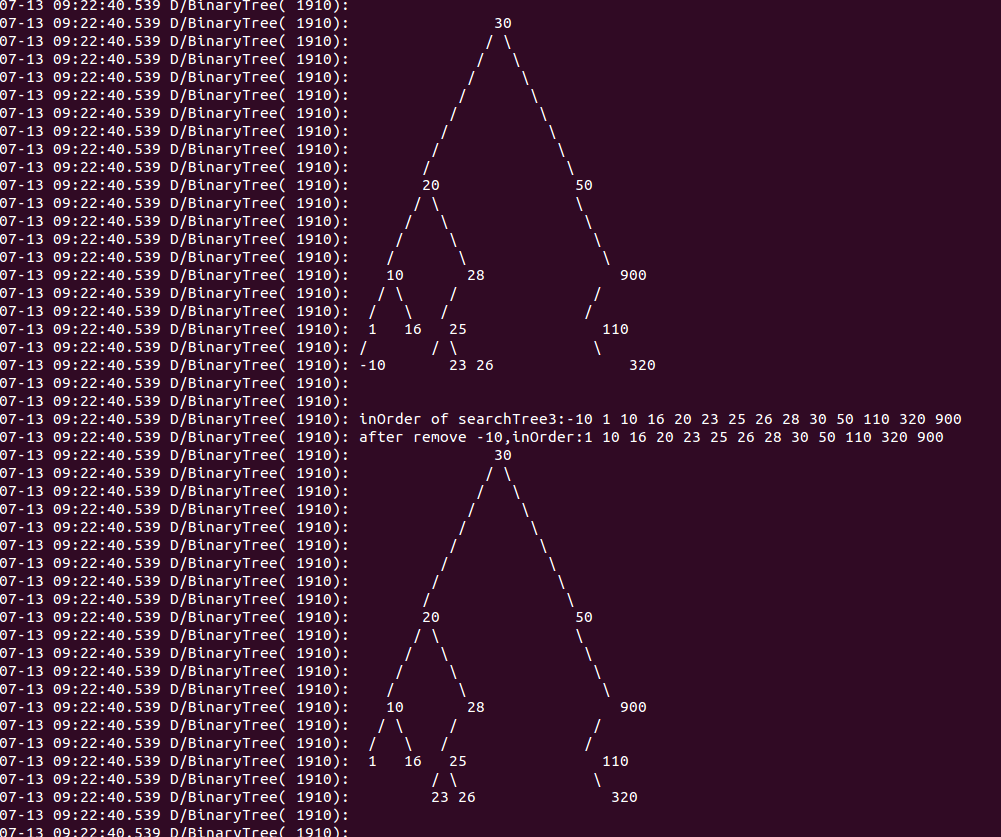
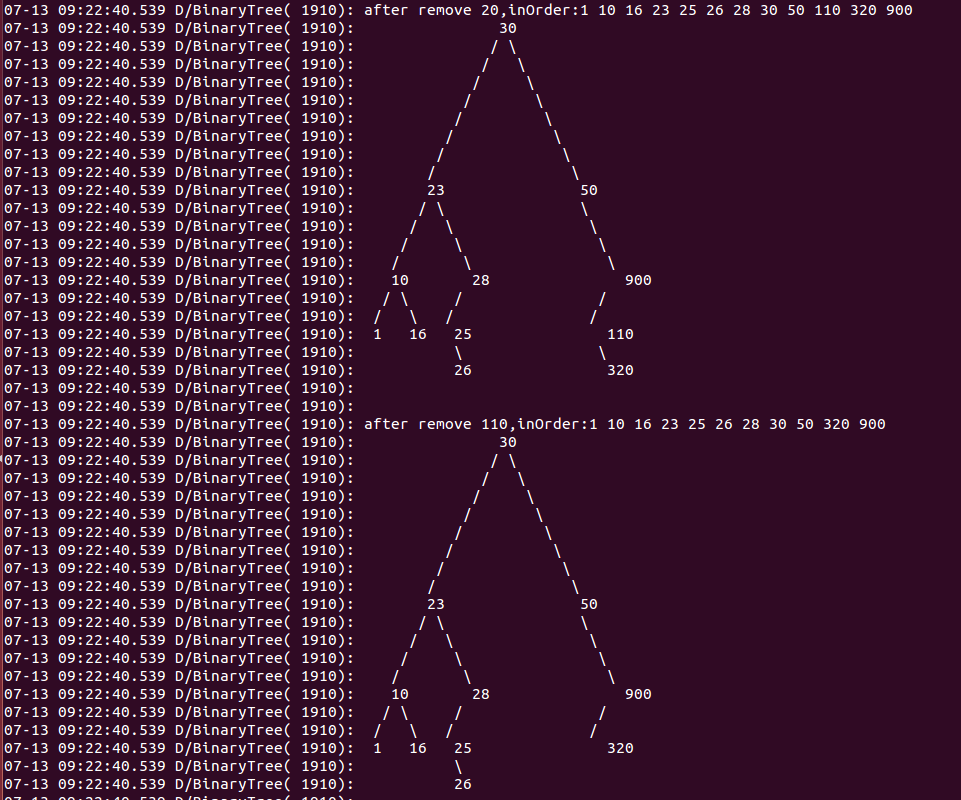