Google推荐的Android架构使用方式
引入
Lifecycles,LiveData,ViewModel
|
|
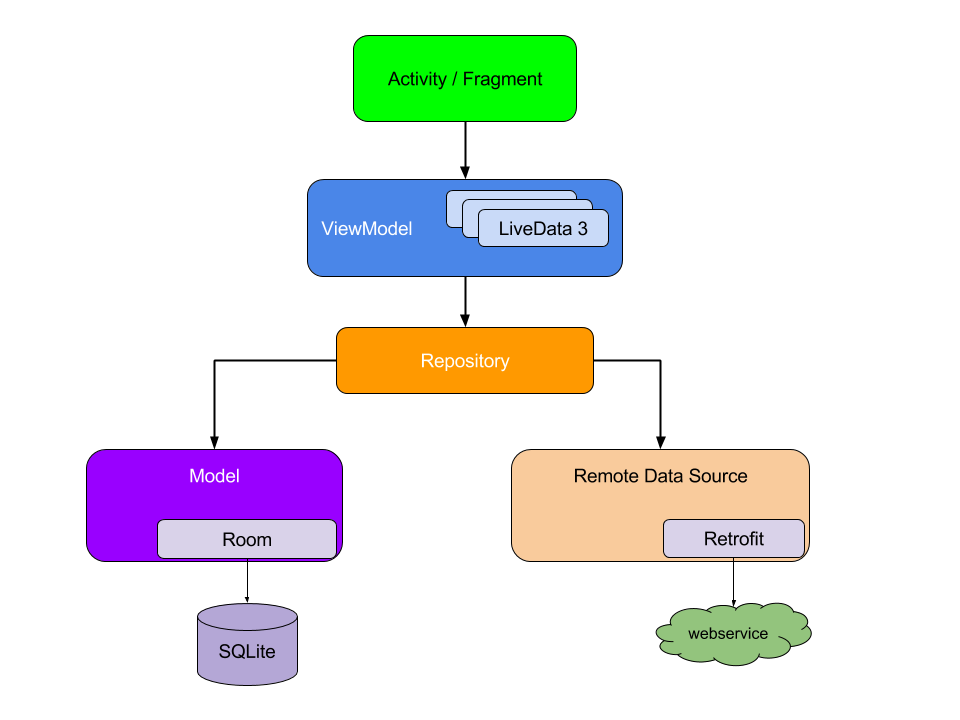
Room
|
|
如果需要使用RoomRxJava,则需要引入
|
|
需要注意的是,这里面使用到了rxjava2,所以如果之前使用了rxjava1的话,会有冲突.
考虑后续rxjava的升级。
LifeCycle
这个其实是这些组件设计的核心。
1.观察主体
观察主体就是Activity和Fragment喽,可以直接继承LifecycleActivity或LifecycleFragment,也可以自己去实现LifecycleRegistry接口。
|
|
实现了LifecyleRegistryOwner之后,就可以充当观察主体了。
2.观察者
只需要实现LifecylceObserver,然后自己对什么事件感兴趣,就自己添加相应的注解。
@LifecycleEvent
如下是一个示例:
|
|
3.注册
在Activity或者Fragment中添加如下代码即可:
|
|
LiveData
LiveData是一个可数据持有者,它相比普通的observable的优势是知道Android组件的生命周期,它只有在Android组件处于活动状态时才会向其发送监听回调。
以定位为例,LocationLiveData可这样写
|
|
使用方式如下
|
|
这样即可在Activity中监听数据的变化,而定位的开始和停止则在LocationLiveData中控制.
不过上面这个例子其实举得不是很好,更常用的是我们在LiveData的外面获取到数据,然后为其setValue即可。在下面ViewModel中就是这样的例子。
ViewModel
ViewModel需要跟LiveData结合起来,这样View就不需要跟Model直接关联,就可以监听到值的变化
ViewModel的好处是:
- 不会在由于configuration改变引起的onDestroy而销毁数据
示意图如下:
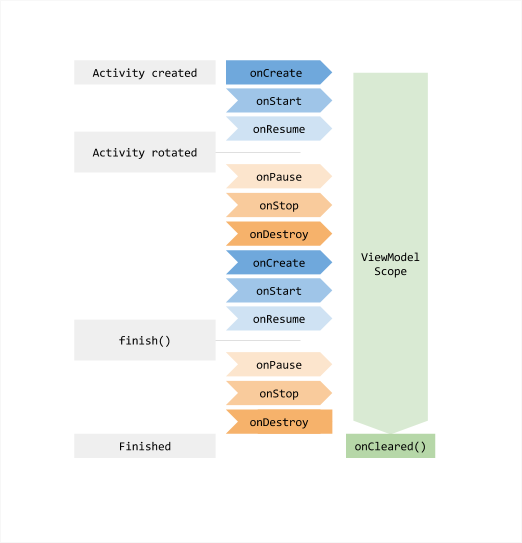
一般来说ViewModel是与LiveData结合起来使用,如下
|
|
在Activity中的使用如下:
|
|
在这里,ViewModel与LiveData结合之后,通过LiveData实现了对于Activity生命周期的监听,所以不会在activity finish之后还收到回调;
同时,由于ViewModel又实现了数据在Activity生命周期内可以一直存活。
Repository
其实就等同于Model,只不过刚刚为了简化,将数据的获取过程由Handler来模拟。
到这里,如果不考虑数据持久化,就可以写出一个比较完整的由LiveData,ViewModel和Repository架构成的例子了。
首先是http相关的接口定义及实现
|
|
然后是UserRepository,网络请求是通过它来调用的
|
|
最后是ViewModel
|
|
在Activity中的使用与前面类似,不再赘述。
Room
Room的作用是通过注解来简化java bean保存到数据库或者从数据库中获取的操作。它主要包含Database,Entity,DAO这3大部分。示意图如下:
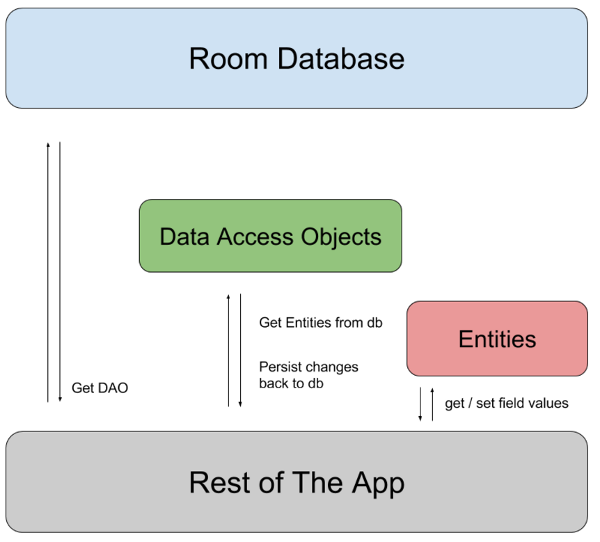
下面举一个简单的例子:
首先是java bean的定义:
|
|
然后是Dao的定义:
|
|
最后是Database的定义
|
|
可以看到,AppDatabase可以对应多个Entity.
最后,对数据库操作进行封装
|
|
到这里,有了UserDao之后,我们就可以写一个带有数据持久化功能的完整示例, 跟前面相比,只需要修改一下UserRepository即可
|
|
高级用法
与Dagger2, RxJava2的结合等